Business - A PHP library for business date calculations
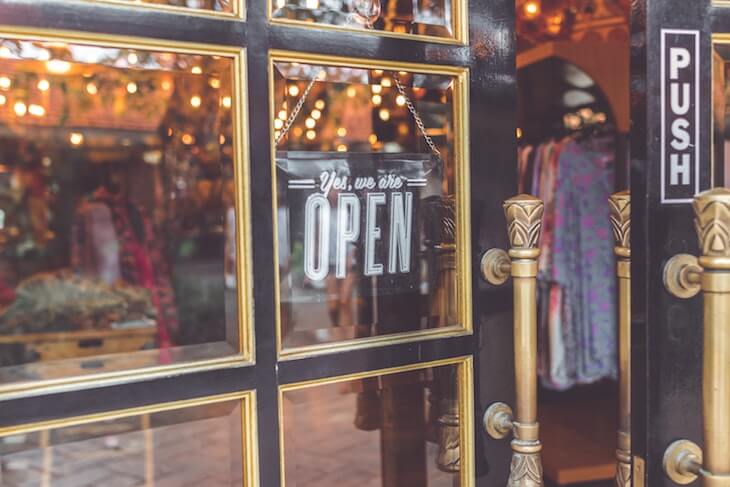
Recently, I released Business, a PHP library to perform business hours date and time calculations.
It can be used in various contexts where working (opening) hours or holidays are involved like bookings, payrolls or time sheets.
With this library you can easily determine booking dates, the number of business days within a given period or the next opening date of your shop.
Installation
To install the latest version with Composer, issue the following command
$ composer require florianv/business
Usage
First, you need to configure a Business
instance before starting to perform calculations.
<?php
use Business\Day;
use Business\Days;
use Business\Business;
use Business\SpecialDay;
// Opening hours for each week day. If not specified, it is considered closed
$days = [
// Standard days with fixed opening hours
new Day(Days::MONDAY, [['09:00', '13:00'], ['2pm', '5 PM']]),
new Day(Days::TUESDAY, [['9 AM', '5 PM']]),
new Day(Days::WEDNESDAY, [['10:00', '13:00'], ['14:00', '17:00']]),
new Day(Days::THURSDAY, [['10 AM', '5 PM']]),
// Special day with dynamic opening hours depending on the date
new SpecialDay(Days::FRIDAY, function (\DateTime $date) {
if ('2015-05-29' === $date->format('Y-m-d')) {
return [['9 AM', '12:00']];
}
return [['9 AM', '5 PM']];
}),
];
// Optional holiday dates
$holidays = [new \DateTime('2015-06-01'), new \DateTime('2015-06-02')];
// Optional business timezone
$timezone = new \DateTimeZone('Europe/Paris');
// Create a new Business instance
$business = new Business($days, $holidays, $timezone);
Operations
Here is the list of the currently implemented operations.
within
- Checks if a date is within business hours
<?php
// This is a Monday and within Monday's opening hours.
$monday = new \DateTime('2015-05-25 09:00');
// As per Friday's special day definition, opening hours are from 09:00 to 12:00 this day
// so this date is not within business hours.
$friday = new \DateTime('2015-05-29 12:01');
// => true
$business->within($monday);
// => false
$business->within($friday);
timeline
- Returns a timeline of business dates
<?php
// Friday 29th May at opening time
$start = new \DateTime('2015-05-29 09:00');
// Monday 29th June at closing time
$end = new \DateTime('2015-06-29 17:00');
// One day interval
$interval = new \DateInterval('P1D');
// Get all business dates during the period
$dates = $business->timeline($start, $end, $interval);
// => 20
$businessDays = count($dates);
As you can see, there are 20 business days during this period (Monday 1st and Tuesday 2nd June being holidays).
closest
- Returns the closest business date from a given date
<?php
$sunday = new \DateTime('2015-05-31');
// The closest date after Sunday is Wednesday at opening time "2015-06-03 10:00:00"
// because Monday and Tuesday are holidays
$closestNext = $business->closest($sunday);
// The closest date before Sunday is Friday at closing time "2015-05-29 12:00:00"
$closestLast = $business->closest($sunday, Business::CLOSEST_LAST);
That's it for now!
If you want to suggest a new feature or report a bug, feel free to do it on the Github repository!